Exploring the Benefits and Limitations of Lazy Loading in EF Core
After a long time without writing about Entity Framework, I will resume with a series of articles about the new version: Entity Framework Core.
For those who still don't know, EF Core is part of the .NET Core, which is cross-platform.
First, we need to understand that EF Core is a new ORM, created literally from ZERO, and for this reason, it still has many things to do! Remember that Entity Framework 6 continues to exist as part of the .NET Full Framework.
In EF Core, with the release of version 2.1, we had a good evolution of the tool, and also the implementation of Lazy Loading
.
But what is Lazy Loading?
When we have relationships in our data model, for example, a Customer
with Order
, EF allows us, when reading the customer, that orders can be accessed as well without using Include
.
But this can make everything very slow, as we can have several customers, with several orders, and the orders also have other relationships, such as products, sellers, etc.
For data loading to be faster, lazy loading is employed and the related data is retrieved only if it is consulted, or triggered.
To demonstrate this in practice, let's create a new Visual Studio Code project console project, and to make this even more fun, let's do everything on the command line:
dotnet new console
dotnet add package Microsoft.EntityFrameworkCore
dotnet add package Microsoft.EntityFrameworkCore.SqlServer
These commands will create a .NET Core
console project and add the EF Core and SQL Server provider.
I will use the Northwind
database for this example (I will put the script on Gist)
Now that we have the project, let's create two classes: Customer
and Order
(If you prefer, you can reverse engineer using this other article):
In this example, I am not using all columns in the tables, as this will not interfere.
And finally our context class:
Well, so far nothing different, right? So let's list the data:
And the result of the execution:
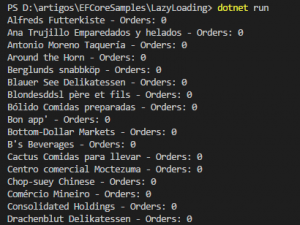
See that all customers have ZERO Orders. That is because Lazy Loading is still disabled!
So let's enable Lazy Loading by adding a new package to our project:
dotnet add package Microsoft.EntityFrameworkCore.Proxies
We can now enable lazy loading in our context with the following code in the Context
class:
using Microsoft.EntityFrameworkCore.Proxies;
and by adding the command:
optionsBuilder.UseLazyLoadingProxies();
Our class will look like this:
Okay, now we will execute it again and we will have the Orders:
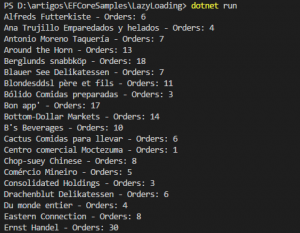
If we compare it to EntityFramework 6, it was a little different, as we only have options for Lazy Loading
and Proxy
, which I don't find particularly interesting, since the resulting object comes with the proxy, see:
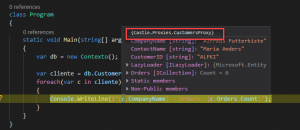
That is because Microsoft used Castle Proxy to implement Lazy Loading
, something that will be improved in later versions.
The source code for this project is on my GitHub at https://github.com/carloscds/EFCoreSamples